EnergiaMedianteVaporAireOGasSevernspdf (April-2022)
- conshalmireti
- May 12, 2022
- 1 min read
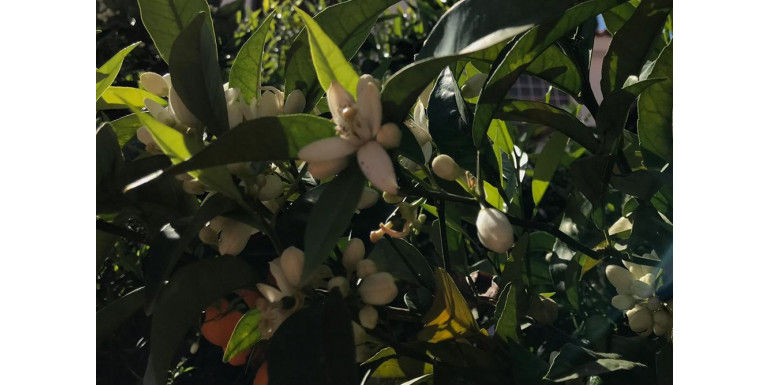
sonlinemaintainfulloperativedpmloginpagelibrarys.Q:
Is it possible to move or copy a texture on the screen from the camera or from another texture?
Is it possible to move or copy a texture on the screen from the camera or from another texture? I could do it using glTexCoordPointer, but it's difficult for me to do because my texture is larger than the screen, so i need to find out if there is any way to do this using openGL.
A:
I believe what you want to do is create a big texture for the whole screen and draw a bunch of smaller ones from it using glTexSubImage2D, but before you do that, I suggest you make a mini-hello-world OpenGL program to get familiar with how it works.
The basic structure is this:
#include
int main(int argc, char *argv[])
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_RGBA | GLUT_DOUBLE);
glutInitWindowSize(320, 480);
glutInitWindowPosition(100, 100);
glutCreateWindow("Hello, GL!");
glutDisplayFunc(myDisplayFunc);
glutIdleFunc(myIdleFunc);
glutMainLoop();
return 0;
}
If you compile that, you should get a window that looks something like this:
And the basic contents of myDisplayFunc (replacing #include and #include with the include files for the actual GL library you're using):
void myDisplayFunc(void)
glClear(GL_COLOR_BUFFER_BIT);
glColor3f(1.0, 1.0, 1.0);
glutPostRedisplay();
If you want to copy part of your big texture onto the screen, you just have to draw some smaller images from the big texture:
#include
Related links:
Comentarios